Wince实现蓝牙设备搜寻
本代码实例实现在windows mobile上搜寻蓝牙设备的功能。
操作环境 :windows mobile系统
SDK :windows mobile 5.0 Pocket PC
主要的API :
WSALookupServiceBegin(
IN LPWSAQUERYSETW lpqsRestrictions,
IN DWORD dwControlFlags,
OUT LPHANDLE lphLookup);
说明:实现搜寻的初始化
WSALookupServiceNext(
IN HANDLE hLookup,
IN DWORD dwControlFlags,
IN OUT LPDWORD lpdwBufferLength,
OUT LPWSAQUERYSETW lpqsResults);
说明:实现搜寻的功能
WSALookupServiceEnd(
IN HANDLE hLookup);
说明:关闭搜寻
Code:
// 本函数实现搜寻蓝牙设备,如果查找到,则名称添加到列表中
void PopulateBtDevList(HWND hDlg)
{
INT iResult = 0;
LPWSAQUERYSET pwsaResults;
DWORD dwSize = 0;
WSAQUERYSET wsaq;
HCURSOR hCurs;
HANDLE hLookup = 0;
// empty the list box
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_RESETCONTENT, 0, 0);
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_ADDSTRING, 0, (LPARAM)_T("Results..."));
memset(&wsaq, 0, sizeof(wsaq));
wsaq.dwSize = sizeof(wsaq);
wsaq.dwNameSpace = NS_BTH;
wsaq.lpcsaBuffer = NULL;
// initialize searching procedure
iResult = WSALookupServiceBegin(&wsaq, LUP_CONTAINERS, &hLookup);
if (iResult != 0)
{
TCHAR tszErr[32];
iResult = WSAGetLastError();
StringCchPrintf(tszErr, 32, _T("Socket Error: %d"), iResult);
MessageBox(hDlg, tszErr, _T("Error"), MB_OK);
}
union {
CHAR buf[5000]; // returned struct can be quite large
SOCKADDR_BTH __unused; // properly align buffer to BT_ADDR requirements
};
// save the current cursor
hCurs = GetCursor();
for (; ;)
{
// set the wait cursor while searching
SetCursor(LoadCursor(NULL, IDC_WAIT));
pwsaResults = (LPWSAQUERYSET)buf;
dwSize = sizeof(buf);
memset(pwsaResults, 0, sizeof(WSAQUERYSET));
pwsaResults->dwSize = sizeof(WSAQUERYSET);
// namespace MUST be NS_BTH for bluetooth queries
pwsaResults->dwNameSpace = NS_BTH;
pwsaResults->lpBlob = NULL;
// iterate through all found devices, returning name and address
// (this sample only uses the name, but address could be used for
// further queries)
iResult = WSALookupServiceNext(hLookup, LUP_RETURN_NAME|LUP_RETURN_ADDR, &dwSize, pwsaResults);
if (iResult != 0)
{
iResult = WSAGetLastError();
if (iResult != WSA_E_NO_MORE)
{
TCHAR tszErr[32];
iResult = WSAGetLastError();
StringCchPrintf(tszErr, 32, _T("Socket Error: %d"), iResult);
MessageBox(hDlg, tszErr, _T("Error"), MB_OK);
}
// we're finished
break;
}
// add the name to the listbox
if (pwsaResults->lpszServiceInstanceName)
{
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_ADDSTRING, 0, (LPARAM)pwsaResults->lpszServiceInstanceName);
}
}
WSALookupServiceEnd(hLookup);
// restore cursor
SetCursor(hCurs);
}
操作环境 :windows mobile系统
SDK :windows mobile 5.0 Pocket PC
主要的API :
WSALookupServiceBegin(
IN LPWSAQUERYSETW lpqsRestrictions,
IN DWORD dwControlFlags,
OUT LPHANDLE lphLookup);
说明:实现搜寻的初始化
WSALookupServiceNext(
IN HANDLE hLookup,
IN DWORD dwControlFlags,
IN OUT LPDWORD lpdwBufferLength,
OUT LPWSAQUERYSETW lpqsResults);
说明:实现搜寻的功能
WSALookupServiceEnd(
IN HANDLE hLookup);
说明:关闭搜寻
Code:
// 本函数实现搜寻蓝牙设备,如果查找到,则名称添加到列表中
void PopulateBtDevList(HWND hDlg)
{
INT iResult = 0;
LPWSAQUERYSET pwsaResults;
DWORD dwSize = 0;
WSAQUERYSET wsaq;
HCURSOR hCurs;
HANDLE hLookup = 0;
// empty the list box
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_RESETCONTENT, 0, 0);
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_ADDSTRING, 0, (LPARAM)_T("Results..."));
memset(&wsaq, 0, sizeof(wsaq));
wsaq.dwSize = sizeof(wsaq);
wsaq.dwNameSpace = NS_BTH;
wsaq.lpcsaBuffer = NULL;
// initialize searching procedure
iResult = WSALookupServiceBegin(&wsaq, LUP_CONTAINERS, &hLookup);
if (iResult != 0)
{
TCHAR tszErr[32];
iResult = WSAGetLastError();
StringCchPrintf(tszErr, 32, _T("Socket Error: %d"), iResult);
MessageBox(hDlg, tszErr, _T("Error"), MB_OK);
}
union {
CHAR buf[5000]; // returned struct can be quite large
SOCKADDR_BTH __unused; // properly align buffer to BT_ADDR requirements
};
// save the current cursor
hCurs = GetCursor();
for (; ;)
{
// set the wait cursor while searching
SetCursor(LoadCursor(NULL, IDC_WAIT));
pwsaResults = (LPWSAQUERYSET)buf;
dwSize = sizeof(buf);
memset(pwsaResults, 0, sizeof(WSAQUERYSET));
pwsaResults->dwSize = sizeof(WSAQUERYSET);
// namespace MUST be NS_BTH for bluetooth queries
pwsaResults->dwNameSpace = NS_BTH;
pwsaResults->lpBlob = NULL;
// iterate through all found devices, returning name and address
// (this sample only uses the name, but address could be used for
// further queries)
iResult = WSALookupServiceNext(hLookup, LUP_RETURN_NAME|LUP_RETURN_ADDR, &dwSize, pwsaResults);
if (iResult != 0)
{
iResult = WSAGetLastError();
if (iResult != WSA_E_NO_MORE)
{
TCHAR tszErr[32];
iResult = WSAGetLastError();
StringCchPrintf(tszErr, 32, _T("Socket Error: %d"), iResult);
MessageBox(hDlg, tszErr, _T("Error"), MB_OK);
}
// we're finished
break;
}
// add the name to the listbox
if (pwsaResults->lpszServiceInstanceName)
{
SendMessage(GetDlgItem(hDlg, IDC_DEVLIST), LB_ADDSTRING, 0, (LPARAM)pwsaResults->lpszServiceInstanceName);
}
}
WSALookupServiceEnd(hLookup);
// restore cursor
SetCursor(hCurs);
}
我来回答
回答0个
时间排序
认可量排序
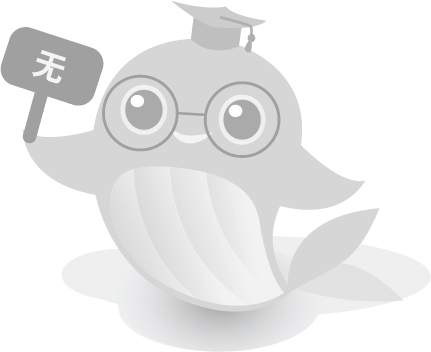
或将文件直接拖到这里
悬赏:
E币
网盘
* 网盘链接:
* 提取码:
悬赏:
E币
Markdown 语法
- 加粗**内容**
- 斜体*内容*
- 删除线~~内容~~
- 引用> 引用内容
- 代码`代码`
- 代码块```编程语言↵代码```
- 链接[链接标题](url)
- 无序列表- 内容
- 有序列表1. 内容
- 缩进内容
- 图片
相关问答
-
2012-12-05 13:32:08
-
2009-11-06 20:23:42
-
2012-12-04 13:21:01
-
2008-08-27 23:38:01
-
2012-12-04 13:58:18
-
2012-12-24 14:14:27
-
2017-08-18 17:24:00
-
2020-05-23 12:04:27
-
2015-11-22 12:39:59
-
32016-12-30 16:55:54
-
2012-12-05 11:10:23
-
2012-12-05 14:28:56
-
2010-01-28 10:58:40
-
2012-12-04 13:13:47
-
2018-12-19 16:01:41
-
2016-04-18 17:35:25
-
2013-08-29 19:28:56
-
2015-03-24 23:52:40
-
2015-03-24 23:53:19
无更多相似问答 去提问

点击登录
-- 积分
-- E币
提问
—
收益
—
被采纳
—
我要提问
切换马甲
上一页
下一页
悬赏问答
-
5hi3516dv500改了sensor驱动后使用pqtools出图出现彩色的竖条纹
-
10海思3559的VGS模块在VI上画线问题
-
53519dv500接lvds的sensor mn34120,图像出现很多竖线,sensor板接以前的3519v101没问题
-
103403外接hdmi口1024*600显示屏报错
-
5SS928点DC camera的6946,全屏紫色
-
5hi3519 的 网络传输的MTU值可以修改到比1500大嘛?
-
10WS73V100星闪扫描不到设备sle
-
5SS928/SD3403 录像失败 venc stream time out, exit thread; venc 2 stream buffer is full
-
10Hi3516DV500无法运行PQTool软件
-
10君正T23+1084带TF卡插卡(给该主板适配TF卡驱动,电机驱动,适配GPIO)
举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
提醒
你的问题还没有最佳答案,是否结题,结题后将扣除20%的悬赏金
取消
确认
提醒
你的问题还没有最佳答案,是否结题,结题后将根据回答情况扣除相应悬赏金(1回答=1E币)
取消
确认