4241
- 收藏
- 点赞
- 分享
- 举报
简单的扫雷的代码
Mini Minesweeper is a simple game in which a user clicks on a square to determ
ine if there is a bomb underneath. If there is, the game is over. If there is
not, they continue clicking until either they find the bomb or all squares (ex
cluding the bomb) have been clicked on.
Write a simple application called MiniMineSweeper that implements this simple
game and creates the GUI.
The application should have the following behaviour:
• At the beginning (and only at the beginning!) of the program the follo
wing dialog should be displayed to the user:
On initialisation, the application should randomly choose where the bomb is lo
cated.
• A square is grey if it has not been clicked on. When a grey square is
clicked, it will:
o Change to white if there is no bomb there.
o Change to black if there is a bomb there.
• The game terminates when only one square is left (and it is the bomb s
quare) or the last square clicked was the bomb. You should inform the user as
to whether or not they have won and ask them if they wish to play again. The
user playing the game in Figure 1 has lost.
上面是要求,一些图片没有弄出来,麻烦,呵呵。
现在简单介绍下如何做的。
先要做一个GUI 。继承JFrame,这样我们就可以显示一个GUI。
我们要生成一个随机种子,用以确定雷点。这里为了简单,我们只有9个方块,其中一个是
雷点。我们用random生成一个值就可以了。
要生成9个按钮,3×3形式的。用GridLayout 方式排列就可以了。
还要监听按钮被触动的事件,如何确定哪个已经被扫过了呢,哪个没有被扫过呢?
哪个是雷呢?
我们可以设置每个按钮的名字就是按钮号,这样我们点了那个按钮,就可以判断出是不是
雷,设置按钮的状态Enabled =false;就可以了。
消息对话框我们可以用JOptionPane.showConfirmDialog,设置就可以了。
大概重要的东西就是上面,我下面贴出了源代码,代码写的有些不规范。呵呵。[code]package com.mine;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class MainFrame extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private JButton jbtn[];//按钮
private int randmValue;//生成的随机值
private static int clickCount = 1;//点击次数
private static int Count = 3*3;//sequare的个数
private JPanel contentPanel;//显示面板
/**
* 设置界面
*
*/
public MainFrame() {
this.setTitle("Boom!");
this.setSize(400, 200);
this.setLocation(400, 300);
initFrame();
this.setVisible(true);
MessageDiag();
this.addWindowListener(new WindowAdapter() {
public void windowClosed(WindowEvent e) {
System.exit(0);
}
public void windowClosing(WindowEvent e){
e.getWindow().dispose();
}
});
}
/**
* 生成一个随机的炸弹的位置1-9
* @param rand
* @return
*/
public int getRandom(int rand) {
int irand = 0;
Random randm = new Random();
irand = randm.nextInt(rand);
System.out.println(irand + 1);
return irand+1;
}
/**
* 创建按钮
* @param count
*/
public void createJbtn(int count) {
jbtn = new JButton[count];
for (int i = 0; i < count; i++) {
jbtn = new JButton();
jbtn.setName(String.valueOf(i + 1));
jbtn.setBackground(Color.gray);
jbtn.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
String msg;
JButton temp = (JButton) ae.getSource();
int name = Integer.parseInt(temp.getName());
if (clickCount < Count - 1) {
if (name != randmValue) {
temp.setBackground(Color.white);
temp.setEnabled(false);
} else {
temp.setBackground(Color.black);
temp.setEnabled(false);
msg="BOOooM!!!";
int iselect = replayYes_No(msg);
if (iselect == 0) {
resetValue(true);
} else{
resetValue(false);
}
}
} else if (clickCount == Count - 1) {
temp.setBackground(Color.white);
msg="congratulation";
int iselect = replayYes_No(msg);
if (iselect == 0) {
resetValue(true);
} else{
resetValue(false);
}
}
clickCount++;
}
});
}
}
/**
* 重玩的时候,
* 重新设置按钮
*
*/
public void resetValue(boolean bool) {
for (int i = 0; i < Count; i++) {
jbtn.setBackground(Color.gray);
jbtn.setEnabled(bool);
}
clickCount = 0;
randmValue = this.getRandom(Count);
}
/**
* 显示初始信息的对话框
*
*/
public void MessageDiag() {
String str = "Welcome to Mini-MiniSweeper.\r\n"
+ "The object of the game is to click on all\r\n"
+ " the squares Except the one with the bomb.\r\n"
+ "(There is only one bomb).To choose a square\r\n"
+ " to display please click on the sequare.\r\n";
JOptionPane.showMessageDialog(this, str, "information",
JOptionPane.INFORMATION_MESSAGE);
}
/**
* 重新玩的显示对话框
*
* @param msg
* @return
*/
public int replayYes_No(String msg ) {
int iselect = JOptionPane.showConfirmDialog(null,
msg+" Would you play again!", "重新玩?",
JOptionPane.YES_NO_OPTION);
return iselect;
}
/**
*初始界面
*
*/
public void initFrame() {
contentPanel = new JPanel(new GridLayout(3, 3));
randmValue = this.getRandom(Count);
createJbtn(Count);
for (int i = 0; i < Count; i++) {
contentPanel.add(jbtn);
}
this.setContentPane(contentPanel);
}
/**
* 主函数
* @param args
*/
public static void main(String args[]) {
new MainFrame();
}
}[/code]
ine if there is a bomb underneath. If there is, the game is over. If there is
not, they continue clicking until either they find the bomb or all squares (ex
cluding the bomb) have been clicked on.
Write a simple application called MiniMineSweeper that implements this simple
game and creates the GUI.
The application should have the following behaviour:
• At the beginning (and only at the beginning!) of the program the follo
wing dialog should be displayed to the user:
On initialisation, the application should randomly choose where the bomb is lo
cated.
• A square is grey if it has not been clicked on. When a grey square is
clicked, it will:
o Change to white if there is no bomb there.
o Change to black if there is a bomb there.
• The game terminates when only one square is left (and it is the bomb s
quare) or the last square clicked was the bomb. You should inform the user as
to whether or not they have won and ask them if they wish to play again. The
user playing the game in Figure 1 has lost.
上面是要求,一些图片没有弄出来,麻烦,呵呵。
现在简单介绍下如何做的。
先要做一个GUI 。继承JFrame,这样我们就可以显示一个GUI。
我们要生成一个随机种子,用以确定雷点。这里为了简单,我们只有9个方块,其中一个是
雷点。我们用random生成一个值就可以了。
要生成9个按钮,3×3形式的。用GridLayout 方式排列就可以了。
还要监听按钮被触动的事件,如何确定哪个已经被扫过了呢,哪个没有被扫过呢?
哪个是雷呢?
我们可以设置每个按钮的名字就是按钮号,这样我们点了那个按钮,就可以判断出是不是
雷,设置按钮的状态Enabled =false;就可以了。
消息对话框我们可以用JOptionPane.showConfirmDialog,设置就可以了。
大概重要的东西就是上面,我下面贴出了源代码,代码写的有些不规范。呵呵。[code]package com.mine;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class MainFrame extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private JButton jbtn[];//按钮
private int randmValue;//生成的随机值
private static int clickCount = 1;//点击次数
private static int Count = 3*3;//sequare的个数
private JPanel contentPanel;//显示面板
/**
* 设置界面
*
*/
public MainFrame() {
this.setTitle("Boom!");
this.setSize(400, 200);
this.setLocation(400, 300);
initFrame();
this.setVisible(true);
MessageDiag();
this.addWindowListener(new WindowAdapter() {
public void windowClosed(WindowEvent e) {
System.exit(0);
}
public void windowClosing(WindowEvent e){
e.getWindow().dispose();
}
});
}
/**
* 生成一个随机的炸弹的位置1-9
* @param rand
* @return
*/
public int getRandom(int rand) {
int irand = 0;
Random randm = new Random();
irand = randm.nextInt(rand);
System.out.println(irand + 1);
return irand+1;
}
/**
* 创建按钮
* @param count
*/
public void createJbtn(int count) {
jbtn = new JButton[count];
for (int i = 0; i < count; i++) {
jbtn = new JButton();
jbtn.setName(String.valueOf(i + 1));
jbtn.setBackground(Color.gray);
jbtn.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent ae) {
String msg;
JButton temp = (JButton) ae.getSource();
int name = Integer.parseInt(temp.getName());
if (clickCount < Count - 1) {
if (name != randmValue) {
temp.setBackground(Color.white);
temp.setEnabled(false);
} else {
temp.setBackground(Color.black);
temp.setEnabled(false);
msg="BOOooM!!!";
int iselect = replayYes_No(msg);
if (iselect == 0) {
resetValue(true);
} else{
resetValue(false);
}
}
} else if (clickCount == Count - 1) {
temp.setBackground(Color.white);
msg="congratulation";
int iselect = replayYes_No(msg);
if (iselect == 0) {
resetValue(true);
} else{
resetValue(false);
}
}
clickCount++;
}
});
}
}
/**
* 重玩的时候,
* 重新设置按钮
*
*/
public void resetValue(boolean bool) {
for (int i = 0; i < Count; i++) {
jbtn.setBackground(Color.gray);
jbtn.setEnabled(bool);
}
clickCount = 0;
randmValue = this.getRandom(Count);
}
/**
* 显示初始信息的对话框
*
*/
public void MessageDiag() {
String str = "Welcome to Mini-MiniSweeper.\r\n"
+ "The object of the game is to click on all\r\n"
+ " the squares Except the one with the bomb.\r\n"
+ "(There is only one bomb).To choose a square\r\n"
+ " to display please click on the sequare.\r\n";
JOptionPane.showMessageDialog(this, str, "information",
JOptionPane.INFORMATION_MESSAGE);
}
/**
* 重新玩的显示对话框
*
* @param msg
* @return
*/
public int replayYes_No(String msg ) {
int iselect = JOptionPane.showConfirmDialog(null,
msg+" Would you play again!", "重新玩?",
JOptionPane.YES_NO_OPTION);
return iselect;
}
/**
*初始界面
*
*/
public void initFrame() {
contentPanel = new JPanel(new GridLayout(3, 3));
randmValue = this.getRandom(Count);
createJbtn(Count);
for (int i = 0; i < Count; i++) {
contentPanel.add(jbtn);
}
this.setContentPane(contentPanel);
}
/**
* 主函数
* @param args
*/
public static void main(String args[]) {
new MainFrame();
}
}[/code]
我来回答
回答0个
时间排序
认可量排序
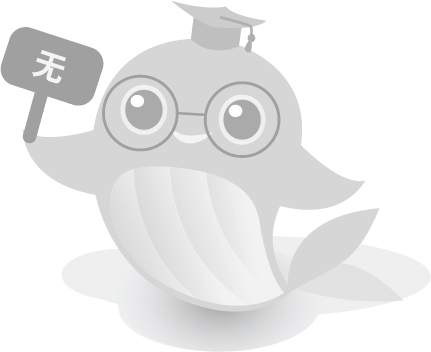
或将文件直接拖到这里
悬赏:
E币
网盘
* 网盘链接:
* 提取码:
悬赏:
E币
Markdown 语法
- 加粗**内容**
- 斜体*内容*
- 删除线~~内容~~
- 引用> 引用内容
- 代码`代码`
- 代码块```编程语言↵代码```
- 链接[链接标题](url)
- 无序列表- 内容
- 有序列表1. 内容
- 缩进内容
- 图片
相关问答
-
2010-01-21 11:12:15
-
2020-11-27 08:58:10
-
22015-09-01 20:25:30
-
2015-08-22 21:22:07
-
2020-08-31 15:28:43
-
2018-12-06 10:26:42
-
2015-06-01 16:14:50
-
2016-04-01 11:29:16
-
2012-11-27 20:41:12
-
2013-11-24 16:41:55
-
2019-12-25 11:34:12
-
2016-10-12 23:02:06
-
2020-11-03 14:22:33
-
2020-01-21 10:12:33
-
2013-08-25 13:11:25
-
102017-12-12 22:48:38
-
2010-01-25 13:54:16
-
2013-11-22 22:18:49
-
2013-11-26 20:25:19
无更多相似问答 去提问

点击登录
-- 积分
-- E币
提问
—
收益
—
被采纳
—
我要提问
切换马甲
上一页
下一页
悬赏问答
-
5SS928的emmc有32GB,bootargs设置使用16GB,但是为啥能用的只有rootfs的大小
-
33SS928怎样烧写ubuntu系统
-
10ToolPlatform下载rootfs提示网络失败
-
10谁有GK7205V500的SDK
-
5Hi3516CV610 烧录不进去
-
10Hi3559AV100 芯片硬解码h265编码格式的视频时出现视频播放错误,解码错误信息 s32PackErr:码流有错
-
5海思SS928 / SD3403的sample_venc.c摄像头编码Demo中,采集到的摄像头的YUV数据在哪个相关的函数中?
-
5海鸥派openEuler无法启动网卡,连接WIFI存在问题
-
66有没有ISP相关的巨佬帮忙看看SS928对接IMX347的图像问题
-
50求助hi3559与FPGA通过SLVS-EC接口对接问题
举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
提醒
你的问题还没有最佳答案,是否结题,结题后将扣除20%的悬赏金
取消
确认
提醒
你的问题还没有最佳答案,是否结题,结题后将根据回答情况扣除相应悬赏金(1回答=1E币)
取消
确认