在鸿蒙上实现简单的语音播报!
在实际应用开发中,时不时的会遇到 AI 领域相关的一些技术,本节主要详细讲述一下语音播报技术,语音播报可能涉及的领域,如:实时语音交互、超长文本播报等。
对于 HarmonyOS 开发者而言,也需要了解和掌握 HarmonyOS AI 领域相关技术能力。
功能介绍
语音播报主要是基于华为智慧引擎(HUAWEI HiAI Engine)中的语音播报引擎,向开发者提供人工智能应用层 API。该技术提供将文本转换为语音并进行播报的能力。
开发指南
①创建与 TTS 服务的连接
context 为应用上下文信息,应为 ohos.aafwk.ability.Ability 或 ohos.aafwk.ability.AbilitySlice 的实例或子类实例。
private static final TtsListener ttsListener = new TtsListener() {
@Override
public void onEvent(int eventType, PacMap pacMap) {
// Log.info("onEvent:" + eventType);
if (eventType == TtsEvent.CREATE_TTS_CLIENT_SUCCESS) {
// Log.info("TTS Client create success");
}
}
@Override
public void onStart(String utteranceId) {
// Log.info(utteranceId + " audio synthesis begins");
}
@Override
public void onProgress(String utteranceId, byte[] audioData, int progress) {
// Log.info(utteranceId + " audio synthesis progress:" + progress);
}
@Override
public void onFinish(String utteranceId) {
// Log.info(utteranceId + " audio synthesis completed");
}
@Override
public void onSpeechStart(String utteranceId) {
// Log.info(utteranceId + " begins to speech");
}
@Override
public void onSpeechProgressChanged(String utteranceId, int progress) {
// Log.info(utteranceId + " speech progress:" + progress);
}
@Override
public void onSpeechFinish(String utteranceId) {
// Log.info(utteranceId + " speech completed");
}
@Override
public void onError(String utteranceId, String errorMessage) {
// Log.info(utteranceId + " errorMessage: " + errorMessage);
}
};
TtsClient.getInstance().create(context, ttsListener);
②在 TTS 接口创建成功后初始化 TTS 引擎:
TtsParams ttsParams = new TtsParams();
ttsParams.setDeviceId("deviceId");
boolean initResult = TtsClient.getInstance().init(ttsParams);
③初始化 TTS 引擎成功后调用音频转换并播放接口:
if (initResult) {
TtsClient.getInstance().speakText("欢迎使用语音播报!", null);
}
④使用完成后销毁 TTS 客户端:
TtsClient.getInstance().destroy();
示例代码
xml 布局:
<?xml version="1.0" encoding="utf-8"?>
<DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:orientation="vertical">
<Text
ohos:height="match_content"
ohos:width="match_content"
ohos:margin="15vp"
ohos:text="AI语音播报"
ohos:text_size="23fp"
ohos:top_margin="40vp"/>
<TextField
ohos:id="$+id:text"
ohos:height="300vp"
ohos:width="match_content"
ohos:layout_alignment="horizontal_center"
ohos:left_margin="20vp"
ohos:multiple_lines="true"
ohos:right_margin="20vp"
ohos:text="某软件公司是中国领先的软件与信息技术服务商,企业数字转型可信赖合作伙伴。公司2001年成立于北京,立足中国,服务全球市场。经过18年发展,目前公司在全球43个城市设有90多个分支机构26个全球交付中心,员工总数近60000人。该软件公司拥有深厚的行业积累和领先的技术实力,可以为客户提供端到端的数字化产品和服务,包括数字化咨询与解决方案、云智能与基础设施、软件与技术服务和数字化运营等;在10余个重要行业服务超过1000家国内外客户,其中世界500强企业客户超过110家,为各领域客户创造价值。"
ohos:text_size="50"
ohos:top_margin="20vp"
/>
<DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:orientation="horizontal">
<Button
ohos:id="$+id:read_btn"
ohos:height="35vp"
ohos:width="80vp"
ohos:background_element="$graphic:background_button"
ohos:margin="15vp"
ohos:text="语音播报"
ohos:text_size="16fp"/>
<Text
ohos:id="$+id:time"
ohos:height="35vp"
ohos:width="150vp"
ohos:margin="15vp"
ohos:text="播报耗时:0 s"
ohos:text_size="16fp"/>
案例代码:
package com.isoftstone.tts.slice;
import com.isoftstone.tts.ResourceTable;
import ohos.aafwk.ability.AbilitySlice;
import ohos.aafwk.content.Intent;
import ohos.agp.components.Button;
import ohos.agp.components.Component;
import ohos.agp.components.Text;
import ohos.agp.components.TextField;
import ohos.ai.tts.TtsClient;
import ohos.ai.tts.TtsListener;
import ohos.ai.tts.TtsParams;
import ohos.ai.tts.constants.TtsEvent;
import ohos.eventhandler.EventHandler;
import ohos.eventhandler.EventRunner;
import ohos.eventhandler.InnerEvent;
import ohos.hiviewdfx.HiLog;
import ohos.hiviewdfx.HiLogLabel;
import ohos.utils.PacMap;
import java.util.Timer;
import java.util.TimerTask;
import java.util.UUID;
public class MainAbilitySlice extends AbilitySlice {
private static final HiLogLabel LABEL_LOG = new HiLogLabel(3, 0xD001100, "MainAbilitySlice");
private TextField infoText;
private Text timeText;
private boolean initItsResult;
private static final int EVENT_MSG_TIME_COUNT = 0x1000002;
private int time = 0;
private Timer timer = null;
private TimerTask timerTask = null;
private EventHandler handler = new EventHandler(EventRunner.current()) {
@Override
protected void processEvent(InnerEvent event) {
switch (event.eventId) {
case EVENT_MSG_TIME_COUNT:
getUITaskDispatcher().delayDispatch(() -> {
time = time + 1;
HiLog.info(LABEL_LOG, "播报耗时:" + time + " s");
timeText.setText("播报耗时:" + time + " s");
}, 0);
break;
default:
break;
}
}
};
@Override
public void onStart(Intent intent) {
super.onStart(intent);
super.setUIContent(ResourceTable.Layout_ability_main);
initView();
initTtsEngine();
}
private void initView() {
infoText = (TextField) findComponentById(ResourceTable.Id_text);
Button readBtn = (Button) findComponentById(ResourceTable.Id_read_btn);
timeText = (Text) findComponentById(ResourceTable.Id_time);
readBtn.setClickedListener(this::readText);
}
private void initTtsEngine() {
TtsClient.getInstance().create(this, ttsListener);
}
private void readText(Component component) {
if (initItsResult) {
TtsParams ttsParams = new TtsParams();
ttsParams.setSpeed(0);//语速0~15越大越快
TtsClient.getInstance().setParams(ttsParams);
HiLog.info(LABEL_LOG, "initItsResult is true, speakText");
TtsClient.getInstance().speakText(infoText.getText(), null);
} else {
HiLog.error(LABEL_LOG, "initItsResult is false");
}
}
private TtsListener ttsListener = new TtsListener() {
@Override
public void onEvent(int eventType, PacMap pacMap) {
HiLog.info(LABEL_LOG, "onEvent...");
// 定义TTS客户端创建成功的回调函数
if (eventType == TtsEvent.CREATE_TTS_CLIENT_SUCCESS) {
TtsParams ttsParams = new TtsParams();
ttsParams.setDeviceId(UUID.randomUUID().toString());
initItsResult = TtsClient.getInstance().init(ttsParams);
}
}
@Override
public void onStart(String utteranceId) {
HiLog.info(LABEL_LOG, "onStart...");
}
@Override
public void onProgress(String utteranceId, byte[] audioData, int progress) {
}
@Override
public void onFinish(String utteranceId) {
HiLog.info(LABEL_LOG, "onFinish...");
}
@Override
public void onError(String s, String s1) {
HiLog.info(LABEL_LOG, "onError...");
}
@Override
public void onSpeechStart(String utteranceId) {
// 开始计时
HiLog.info(LABEL_LOG, "onSpeechStart...");
if (timer == null && timerTask == null) {
timer = new Timer();
timerTask = new TimerTask() {
public void run() {
handler.sendEvent(EVENT_MSG_TIME_COUNT);
}
};
timer.schedule(timerTask, 0, 1000);
}
}
@Override
public void onSpeechProgressChanged(String utteranceId, int progress) {
}
@Override
public void onSpeechFinish(String utteranceId) {
// 结束计时
HiLog.info(LABEL_LOG, "onSpeechFinish...");
timer.cancel();
time = 0;
timer = null;
timerTask = null;
}
};
}
实现效果
如下图:
来源:鸿蒙技术社区
- 分享
- 举报
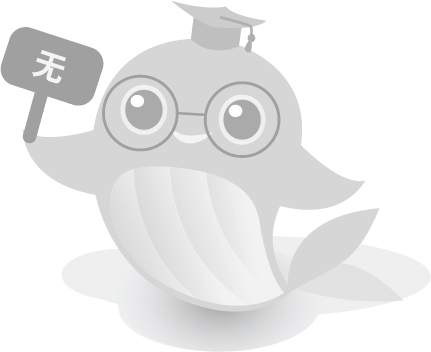
-
浏览量:4777次2021-09-08 16:03:36
-
浏览量:4641次2021-09-26 15:34:36
-
浏览量:7122次2021-07-07 13:43:12
-
浏览量:4238次2021-08-30 16:19:50
-
浏览量:4518次2021-09-23 13:51:06
-
浏览量:5635次2021-08-04 13:46:28
-
浏览量:4266次2021-08-20 16:38:06
-
浏览量:1169次2023-04-18 09:14:22
-
浏览量:624次2023-09-18 14:26:23
-
浏览量:4580次2021-08-17 16:28:57
-
浏览量:4345次2021-08-31 13:39:07
-
浏览量:1510次2023-04-13 10:45:45
-
浏览量:3433次2022-09-03 09:03:21
-
浏览量:4833次2021-06-28 15:59:34
-
浏览量:293次2024-08-23 14:29:14
-
浏览量:2137次2019-12-28 10:40:42
-
浏览量:4343次2021-06-30 09:47:29
-
浏览量:677次2023-09-11 13:55:15
-
浏览量:1346次2023-03-13 18:05:45
-
广告/SPAM
-
恶意灌水
-
违规内容
-
文不对题
-
重复发帖
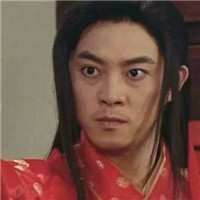
AI算法识别






举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明