技术专栏
qt图表库qcustomplot使用心得记录四 (参考线)
qt图表库qcustomplot使用心得记录四 (参考线)
本系列讲述的是我使用qt图表库qcustomplot中的使用心得分享,借此记录我的学习内容,也希望可以给与初学者一些帮助。
本篇文章讲述的是实现标记线的功能,即使水平线和垂直线,标记线可以在图表内随意拖动。
实现原理
标记线功能的实现原理也不是很难,就是使用绘图在Widget内绘制一条水平/垂直线,在通过鼠标事件使其可以左后/上下拖动,以用来标记一个点。
代码实现:代码中只实现了垂直参考线,水平参考线实现原理同理在这里就不做重复解释了。
#ifndef CUSTONPLOTEX_H
#define CUSTONPLOTEX_H
#include <QWidget>
#include "QCustomPlot/qcustomplot.h"
#include <QRubberBand>
#include "stdio.h"
class CustomPlotEx : public QCustomPlot
{
Q_OBJECT
public:
CustomPlotEx();
CustomPlotEx(QWidget *widget);
~CustomPlotEx() Q_DECL_OVERRIDE;
//锁定,解锁
void lock();
void unLock();
/**********************************选点************************************************/
void showVLine();//显示垂直线 根据垂直线选点
void enableVScratchLine(bool enable);//使能标记线
void enableVScratchLine(bool enable,double key);//使能标记线 (使能标志,标记线位置)
bool vStcratchLineStatus(){return enableSLine;}
double keyFromVScratchLine();
protected:
//键盘按下事件
virtual void keyPressEvent(QKeyEvent *event) Q_DECL_OVERRIDE;
virtual void mousePressEvent(QMouseEvent *event) Q_DECL_OVERRIDE;
virtual void mouseDoubleClickEvent(QMouseEvent *event) Q_DECL_OVERRIDE;
virtual void mouseMoveEvent(QMouseEvent *event) Q_DECL_OVERRIDE;
virtual void mouseReleaseEvent(QMouseEvent *event) Q_DECL_OVERRIDE;
virtual void wheelEvent(QWheelEvent *event) Q_DECL_OVERRIDE;
virtual void paintEvent(QPaintEvent *event) Q_DECL_OVERRIDE;
public slots:
//鼠标按下
void mousePress(QMouseEvent *event);
//鼠标移动
void mouseMove(QMouseEvent *event);
//鼠标释放
void mouseRelease(QMouseEvent *event);
//鼠标滑轮
void mouseWheel(QWheelEvent *event);
private:
void Init();
bool isLock = false;//锁定
bool enableSLine = false;//标记线使能标志
struct LINESEG{
bool bdraw;
bool bselLine;
QPoint startPoint;
QPoint endPoint;
};
QList<LINESEG> lineSegList;
};
#endif // CUSTONPLOTEX_H
#include "customplotex.h"
CustomPlotEx::CustomPlotEx() : QCustomPlot(new QWidget())
{
Init();
}
CustomPlotEx::CustomPlotEx(QWidget *widget) : QCustomPlot(widget)
{
Init();
}
CustomPlotEx::~CustomPlotEx()
{
}
void CustomPlotEx::lock()
{
isLock = true;
}
void CustomPlotEx::unLock()
{
isLock = false;
}
void CustomPlotEx::showVLine()
{
// lineSegList[0].startPoint
lineSegList[0].startPoint.ry() = 0;
lineSegList[0].startPoint.rx() = this->width()/2;
lineSegList[0].endPoint.ry() = this->height();
lineSegList[0].endPoint.rx() = this->width()/2;
lineSegList[0].bdraw = true;
update();
}
void CustomPlotEx::enableVScratchLine(bool enable)
{
enableSLine = enable;
if(enableSLine)
{
lineSegList[0].startPoint.ry() = 0;
lineSegList[0].startPoint.rx() = this->width()/2;
lineSegList[0].endPoint.ry() = this->height();
lineSegList[0].endPoint.rx() = this->width()/2;
lineSegList[0].bdraw = true;
update();
}else{
lineSegList[0].bdraw = false;
update();
}
}
void CustomPlotEx::enableVScratchLine(bool enable, double key)
{
enableSLine = enable;
if(enableSLine)
{
lineSegList[0].startPoint.ry() = 0;
lineSegList[0].startPoint.rx() = static_cast<int>(xAxis->coordToPixel(key));
lineSegList[0].endPoint.ry() = this->height();
lineSegList[0].endPoint.rx() = static_cast<int>(xAxis->coordToPixel(key));
lineSegList[0].bdraw = true;
update();
}else{
lineSegList[0].bdraw = false;
update();
}
}
double CustomPlotEx::keyFromVScratchLine()
{
return xAxis->pixelToCoord(lineSegList.at(0).startPoint.x());
}
void CustomPlotEx::keyPressEvent(QKeyEvent *event)
{
if(isLock)
return;
}
void CustomPlotEx::mousePressEvent(QMouseEvent *event)
{
if(isLock)
return;
if(enableSLine)
{
if(lineSegList[0].bdraw || lineSegList[1].bdraw)
{
lineSegList[0].startPoint.rx() = event->pos().x();
lineSegList[0].endPoint.rx() = event->pos().x();
lineSegList[0].bselLine = true;
return;
}
}
QCustomPlot::mousePressEvent(event);
}
void CustomPlotEx::mouseDoubleClickEvent(QMouseEvent *event)
{
if(isLock)
return;
QCustomPlot::mouseDoubleClickEvent(event);
}
void CustomPlotEx::mouseMoveEvent(QMouseEvent *event)
{
if(isLock)
return;
QCustomPlot::mouseMoveEvent(event);
}
void CustomPlotEx::mouseReleaseEvent(QMouseEvent *event)
{
if(isLock)
return;
QCustomPlot::mouseReleaseEvent(event);
}
void CustomPlotEx::wheelEvent(QWheelEvent *event)
{
if(isLock)
return;
QCustomPlot::wheelEvent(event);
}
void CustomPlotEx::paintEvent(QPaintEvent *event)
{
QCustomPlot::paintEvent(event);
QPainter p(this);
QPen pen;
pen.setColor(QColor(87,152,252));
pen.setWidth(2);
p.setPen(pen);
foreach(auto i,lineSegList)
{
if(i.bdraw)
p.drawLine(i.startPoint,i.endPoint);
}
}
void CustomPlotEx::mousePress(QMouseEvent *event)
{
Q_UNUSED(event);
}
void CustomPlotEx::mouseMove(QMouseEvent *event)
{
if(enableSLine){
if(lineSegList[0].bdraw || lineSegList[1].bdraw)
{
if(lineSegList[0].bselLine)
{
lineSegList[0].startPoint.rx() = event->pos().x();
lineSegList[0].endPoint.rx() = event->pos().x();
update();
return;
}else{
if(event->pos().x() == lineSegList[0].startPoint.x())
{
setCursor(Qt::PointingHandCursor);
return;
}
}
}
}
QList<QCPLayerable*> candidates = layerableListAt(event->pos(), false);
if(candidates.contains(this->xAxis))
{
setCursor(Qt::SizeHorCursor);
}else if(candidates.contains(this->yAxis))
{
setCursor(Qt::SizeVerCursor);
}else{
setCursor(Qt::ArrowCursor);
}
}
void CustomPlotEx::mouseRelease(QMouseEvent *event)
{
if(lineSegList[0].bselLine)
lineSegList[0].bselLine = false;
}
void CustomPlotEx::mouseWheel(QWheelEvent *event)
{
Q_UNUSED(event);
}
void CustomPlotEx::Init()
{
// setAntialiasedElement(QCP::aeAll);//抗锯齿
setInteractions(QCP::iRangeDrag | QCP::iRangeZoom | QCP::iSelectAxes |
QCP::iSelectLegend | QCP::iSelectPlottables);
setPlottingHints(QCP::phFastPolylines | QCP::phImmediateRefresh | QCP::phCacheLabels);
this->setOpenGl(true);
setNoAntialiasingOnDrag(true);
LINESEG vLine;
vLine.bdraw = false;
vLine.bselLine = false;
vLine.startPoint.ry() = 0;
vLine.startPoint.rx() = this->width()/2;
vLine.endPoint.ry() = this->height();
vLine.endPoint.rx() = this->width()/2;
LINESEG hLine;
hLine.bdraw = false;
hLine.bselLine = false;
hLine.startPoint.ry() = 0;
hLine.startPoint.rx() = this->width()/2;
hLine.endPoint.ry() = this->height();
hLine.endPoint.rx() = this->width()/2;
lineSegList.append(vLine);
lineSegList.append(hLine);
connect(this, SIGNAL(mousePress(QMouseEvent*)), this, SLOT(mousePress(QMouseEvent*)));
connect(this, SIGNAL(mouseMove(QMouseEvent*)), this, SLOT(mouseMove(QMouseEvent*)));
connect(this, SIGNAL(mouseRelease(QMouseEvent*)), this, SLOT(mouseRelease(QMouseEvent*)));
connect(this, SIGNAL(mouseWheel(QWheelEvent*)), this, SLOT(mouseWheel(QWheelEvent*)));
}
声明:本文内容由易百纳平台入驻作者撰写,文章观点仅代表作者本人,不代表易百纳立场。如有内容侵权或者其他问题,请联系本站进行删除。
红包
点赞
1
评论
打赏
- 分享
- 举报
评论
0个
手气红包
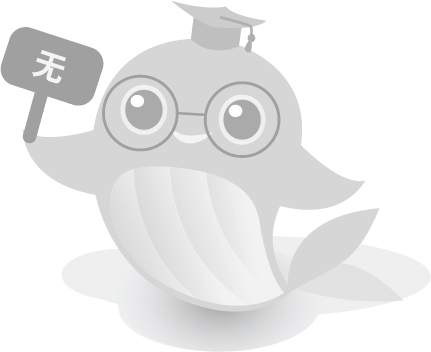
相关专栏
-
浏览量:5556次2020-08-23 21:17:12
-
浏览量:5315次2020-08-23 21:07:51
-
浏览量:2829次2020-08-23 20:59:44
-
浏览量:5038次2020-05-08 15:46:11
-
浏览量:7613次2020-12-11 16:08:02
-
浏览量:3385次2020-05-07 19:43:16
-
浏览量:6635次2018-01-22 14:23:15
-
浏览量:2688次2020-04-24 17:44:09
-
浏览量:1925次2020-08-28 16:40:19
-
浏览量:5136次2020-12-19 17:48:05
-
浏览量:4724次2021-08-10 14:24:09
-
浏览量:4917次2020-09-20 21:47:25
-
浏览量:5727次2021-03-31 15:36:17
-
浏览量:4448次2020-07-31 13:45:09
-
浏览量:819次2023-06-15 11:42:45
-
浏览量:11167次2021-07-13 16:37:15
-
浏览量:8325次2020-12-09 23:14:18
-
浏览量:2918次2020-05-06 15:52:54
-
浏览量:828次2023-12-11 16:43:29
置顶时间设置
结束时间
删除原因
-
广告/SPAM
-
恶意灌水
-
违规内容
-
文不对题
-
重复发帖
打赏作者

小王子🤴
您的支持将鼓励我继续创作!
打赏金额:
¥1

¥5

¥10

¥50

¥100

支付方式:

举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
审核成功
发布时间设置
发布时间:
请选择发布时间设置
是否关联周任务-专栏模块
审核失败
失败原因
请选择失败原因
备注
请输入备注