技术专栏
海思平台的前景提取实现
代码的主要思路是 上上帧 和 上一帧 相减得到 差值图像1,上一帧 和 当前帧 相减得到 差值图像2。差值图像1 与 2 做运算得到前景图像。
/************************************************************
FileName: sample_ive_sfd.c
Author: Merz
Description: frame subtract to extract the foreground image
Version: 1.0
Function List:
1. SAMPLE_IVE_Sub
2. SAMPLE_IVE_Thresh
3. SAMPLE_IVE_Dilate
4. SAMPLE_IVE_And
History:
<author> <time> <version> <desc>
Merz 19/11/4 1.0 build this file
Merz 19/11/6 1.0 complete four functions
***********************************************************/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#include <signal.h>
#include <semaphore.h>
#include <pthread.h>
#include <sys/prctl.h>
#include <time.h>
#include "sample_comm_ive.h"
#define SAMPLE_IVE_SFD_IMAGE_NUM 2 //three frame subtract
typedef struct hiSAMPLE_IVE_SFD_S
{
IVE_SRC_IMAGE_S astSrc[SAMPLE_IVE_SFD_IMAGE_NUM]; //input frame
IVE_SRC_IMAGE_S stImg[SAMPLE_IVE_SFD_IMAGE_NUM]; //diff img
IVE_DST_IMAGE_S stDst; //output frame
FILE* pFpSrc; //read from pFpSrc
FILE* pFpDst; //write to pFpDst
}SAMPLE_IVE_SFD_S;
static SAMPLE_IVE_SFD_S s_stSfd;
static HI_VOID SAMPLE_IVE_Sfd_Uninit(SAMPLE_IVE_SFD_S *pstSfd)
{
HI_U16 i;
for (i = 0; i < 2; i++)
{
IVE_MMZ_FREE(pstSfd->astSrc[i].au64PhyAddr[0], pstSfd->astSrc[i].au64VirAddr[0]);
IVE_MMZ_FREE(pstSfd->stImg[i].au64PhyAddr[0], pstSfd->stImg[i].au64VirAddr[0]);
}
IVE_MMZ_FREE(pstSfd->stDst.au64PhyAddr[0], pstSfd->stDst.au64VirAddr[0]);
IVE_CLOSE_FILE(pstSfd->pFpSrc);
IVE_CLOSE_FILE(pstSfd->pFpDst);
}
static HI_S32 SAMPLE_IVE_Sfd_Init(SAMPLE_IVE_SFD_S *pstSfd, HI_U32 u32Width, HI_U32 u32Height, HI_CHAR *pchSrcFileName, HI_CHAR *pchDstFileName)
{
HI_S32 s32Ret = HI_SUCCESS;
HI_U16 i;
memset(pstSfd, 0, sizeof(SAMPLE_IVE_SFD_S));
for (i = 0; i < 2; i++)
{
s32Ret = SAMPLE_COMM_IVE_CreateImage(&(pstSfd->astSrc[i]), IVE_IMAGE_TYPE_U8C1, u32Width, u32Height);
SAMPLE_CHECK_EXPR_GOTO(HI_SUCCESS != s32Ret, SFD_INIT_FAIL, "Error(%#x),Create src[%d] image failed!\n", s32Ret, i);
s32Ret = SAMPLE_COMM_IVE_CreateImage(&(pstSfd->stImg[i]), IVE_IMAGE_TYPE_U8C1, u32Width, u32Height);
SAMPLE_CHECK_EXPR_GOTO(HI_SUCCESS != s32Ret, SFD_INIT_FAIL, "Error(%#x),Create stImg[%d] image failed!\n", s32Ret, i);
}
s32Ret = SAMPLE_COMM_IVE_CreateImage(&(pstSfd->stDst), IVE_IMAGE_TYPE_U8C1, u32Width, u32Height);
SAMPLE_CHECK_EXPR_GOTO(HI_SUCCESS != s32Ret, SFD_INIT_FAIL, "Error(%#x),Create stDst image failed!\n", s32Ret);
s32Ret = HI_FAILURE;
pstSfd->pFpSrc = fopen(pchSrcFileName, "rb");
SAMPLE_CHECK_EXPR_GOTO(NULL == pstSfd->pFpSrc, SFD_INIT_FAIL, "Error,Open file %s failed!\n", pchSrcFileName);
pstSfd->pFpDst = fopen(pchDstFileName, "wb");
SAMPLE_CHECK_EXPR_GOTO(NULL == pstSfd->pFpDst, SFD_INIT_FAIL, "Error,Open file %s failed!\n", pchDstFileName);
s32Ret = HI_SUCCESS;
SFD_INIT_FAIL:
if (HI_SUCCESS != s32Ret)
{
SAMPLE_IVE_Sfd_Uninit(pstSfd);
}
return s32Ret;
}
/*************************************************
Function: SAMPLE_IVE_Sub
Description: create two gray image subtraction task
Input: pstCurImg: type(U8C1) size(64x64~1920x1080)
pstLastImg: type(U8C1) size(64x64~1920x1080)
Output: pstDiffImg: type(U8C1) size(64x64~1920x1080)
Return: s32Ret
Others:
*************************************************/
static HI_S32 SAMPLE_IVE_Sub(IVE_SRC_IMAGE_S *pstCurImg, IVE_SRC_IMAGE_S *pstLastImg, IVE_DST_IMAGE_S *pstDiffImg)
{
HI_S32 s32Ret;
IVE_HANDLE IveHandle;
IVE_SUB_CTRL_S stSubCtrl;
memset(&stSubCtrl, 0, sizeof(stSubCtrl));
stSubCtrl.enMode = IVE_SUB_MODE_ABS;
s32Ret = HI_MPI_IVE_Sub(&IveHandle, pstCurImg, pstLastImg, pstDiffImg, &stSubCtrl, HI_FALSE);
SAMPLE_CHECK_EXPR_RET(HI_SUCCESS != s32Ret,HI_FALSE,"Error(%#x),HI_MPI_IVE_Sub failed!\n",s32Ret);
return s32Ret;
}
/*************************************************
Function: SAMPLE_IVE_Thresh
Description: create a gray image threshold tasks
Input: pstInImg: type(U8C1) size(64x64~1920x1080)
Output: pstOutImg: type(U8C1) size(64x64~1920x1080)
Return: s32Ret
Others:
*************************************************/
static HI_S32 SAMPLE_IVE_Thresh(IVE_SRC_IMAGE_S *pstInImg, IVE_DST_IMAGE_S *pstOutImg)
{
HI_S32 s32Ret;
IVE_HANDLE IveHandle;
IVE_THRESH_CTRL_S stCtrl;
memset(&stCtrl, 0, sizeof(stCtrl));
stCtrl.enMode = IVE_THRESH_MODE_BINARY;
stCtrl.u8MinVal = 0;
stCtrl.u8MaxVal = 255;
stCtrl.u8LowThr = 35;
s32Ret = HI_MPI_IVE_Thresh(&IveHandle, pstInImg, pstOutImg, &stCtrl, HI_FALSE);
SAMPLE_CHECK_EXPR_RET(HI_SUCCESS != s32Ret,s32Ret,"Error(%#x),HI_MPI_IVE_Thresh failed!\n",s32Ret);
return s32Ret;
}
/*************************************************
Function: SAMPLE_IVE_Dilate
Description: create a binary image 5x5 template dilate tasks
Input: pstInImg: type(U8C1) size(64x64~1920x1024)
Output: pstOutImg: type(U8C1) size(64x64~1920x1024)
Return: s32Ret
Others: template coefficient can only to 0 or 255
*************************************************/
static HI_S32 SAMPLE_IVE_Dilate(IVE_SRC_IMAGE_S *pstInImg, IVE_DST_IMAGE_S *pstOutImg)
{
HI_S32 s32Ret;
IVE_HANDLE IveHandle;
IVE_DILATE_CTRL_S stDilateCtrl;
memset(&stDilateCtrl, 0, sizeof(stDilateCtrl));
memset(&stDilateCtrl.au8Mask, 255, 25);
s32Ret = HI_MPI_IVE_Dilate(&IveHandle, pstInImg, pstOutImg, &stDilateCtrl, HI_FALSE);
SAMPLE_CHECK_EXPR_RET(HI_SUCCESS != s32Ret,s32Ret,"Error(%#x),HI_MPI_IVE_Dilate failed!\n",s32Ret);
return s32Ret;
}
/*************************************************
Function: SAMPLE_IVE_And
Description: create two binary image add tasks
Input: stImg1: type(U8C1) size(64x64~1920x1080)
stImg2: type(U8C1) size(64x64~1920x1080)
Output: stDst: type(U8C1) size(64x64~1920x1080)
Return: s32Ret
Others:
*************************************************/
static HI_S32 SAMPLE_IVE_And(IVE_IMAGE_S *stImg1, IVE_IMAGE_S *stImg2, IVE_DST_IMAGE_S *stDst)
{
HI_S32 s32Ret;
IVE_HANDLE IveHandle;
s32Ret = HI_MPI_IVE_And(&IveHandle, stImg1, stImg2, stDst, HI_FALSE);
SAMPLE_CHECK_EXPR_RET(HI_SUCCESS != s32Ret,s32Ret,"Error(%#x),HI_MPI_IVE_And failed!\n",s32Ret);
return s32Ret;
}
static HI_S32 SAMPLE_IVE_SfdProc(SAMPLE_IVE_SFD_S *pstSfd)
{
HI_S32 s32Ret = HI_SUCCESS;
HI_U32 u32FrmNum;
HI_S32 s32CurIdx = 0;
HI_U32 u32TotalFrm = 200;
HI_BOOL TimeFlag = HI_TRUE;
clock_t start, finish;
double totaltime;
for (u32FrmNum = 1; u32FrmNum < u32TotalFrm; u32FrmNum++)
{
SAMPLE_PRT("Proc Frame %d/%d\n",u32FrmNum,u32TotalFrm);
s32Ret = SAMPLE_COMM_IVE_ReadFile(&(pstSfd->astSrc[s32CurIdx]), pstSfd->pFpSrc);
SAMPLE_CHECK_EXPR_RET(HI_SUCCESS != s32Ret,s32Ret,"Error(%#x),Read src1 file failed!\n",s32Ret);
if (u32FrmNum > 1)
{
if(TimeFlag = HI_TRUE)
{
start = clock();
TimeFlag = HI_FALSE;
}
s32Ret = SAMPLE_IVE_Sub(&(pstSfd->astSrc[s32CurIdx]), &(pstSfd->astSrc[1 - s32CurIdx]), &(pstSfd->stImg[s32CurIdx]));
s32Ret = SAMPLE_IVE_Thresh(&(pstSfd->stImg[s32CurIdx]), &(pstSfd->stImg[s32CurIdx]));
s32Ret = SAMPLE_IVE_Dilate(&(pstSfd->stImg[s32CurIdx]), &(pstSfd->stImg[s32CurIdx]));
if(u32FrmNum > 2)
{
s32Ret = SAMPLE_IVE_And(&(pstSfd->stImg[s32CurIdx]), &(pstSfd->stImg[1 - s32CurIdx]), &pstSfd->stDst);
finish = clock();
totaltime = (double)(finish - start) / CLOCKS_PER_SEC;
printf("Time passed in seconds: %f\n", totaltime);
TimeFlag = HI_TRUE;
s32Ret = SAMPLE_COMM_IVE_WriteFile(&pstSfd->stDst, pstSfd->pFpDst);
}
}
s32CurIdx = 1 - s32CurIdx; //change cur image to last image
}
return s32Ret;
}
HI_VOID SAMPLE_IVE_Sfd(HI_VOID)
{
HI_S32 s32Ret = HI_SUCCESS;
HI_U32 u32Width = 1280;
HI_U32 u32Height = 720;
HI_CHAR pchSrcFileName[IVE_FILE_NAME_LEN];
HI_CHAR pchDstFileName[IVE_FILE_NAME_LEN];
snprintf(pchSrcFileName, sizeof(pchSrcFileName), "./data/input/img/smoke_720p.yuv");
snprintf(pchDstFileName, sizeof(pchDstFileName), "./data/output/sfd/output.yuv");
memset(&s_stSfd,0,sizeof(s_stSfd));
SAMPLE_COMM_IVE_CheckIveMpiInit();
s32Ret = SAMPLE_IVE_Sfd_Init(&s_stSfd, u32Width, u32Height, pchSrcFileName, pchDstFileName);
SAMPLE_CHECK_EXPR_GOTO(HI_SUCCESS != s32Ret, SFD_FAIL, "Error(%#x),SAMPLE_IVE_Sfd_Init failed!\n", s32Ret);
s32Ret = SAMPLE_IVE_SfdProc(&s_stSfd);
if (HI_SUCCESS == s32Ret)
{
SAMPLE_PRT("Process success!\n");
}
SAMPLE_IVE_Sfd_Uninit(&s_stSfd);
memset(&s_stSfd,0,sizeof(s_stSfd));
SFD_FAIL:
SAMPLE_COMM_IVE_IveMpiExit();
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
- 142
- 143
- 144
- 145
- 146
- 147
- 148
- 149
- 150
- 151
- 152
- 153
- 154
- 155
- 156
- 157
- 158
- 159
- 160
- 161
- 162
- 163
- 164
- 165
- 166
- 167
- 168
- 169
- 170
- 171
- 172
- 173
- 174
- 175
- 176
- 177
- 178
- 179
- 180
- 181
- 182
- 183
- 184
- 185
- 186
- 187
- 188
- 189
- 190
- 191
- 192
- 193
- 194
- 195
- 196
- 197
- 198
- 199
- 200
- 201
- 202
- 203
- 204
- 205
- 206
- 207
- 208
- 209
- 210
- 211
- 212
- 213
- 214
- 215
- 216
- 217
- 218
- 219
- 220
- 221
- 222
- 223
- 224
- 225
- 226
- 227
- 228
- 229
- 230
- 231
- 232
- 233
- 234
- 235
- 236
- 237
- 238
- 239
- 240
- 241
- 242
- 243
- 244
- 245
- 246
- 247
- 248
- 249
- 250
- 251
- 252
- 253
- 254
- 255
- 256
- 257
- 258
- 259
- 260
- 261
- 262
- 263
- 264
- 265
- 266
- 267
- 268
- 269
<
声明:本文内容由易百纳平台入驻作者撰写,文章观点仅代表作者本人,不代表易百纳立场。如有内容侵权或者其他问题,请联系本站进行删除。
红包
点赞
收藏
评论
打赏
- 分享
- 举报
评论
0个
手气红包
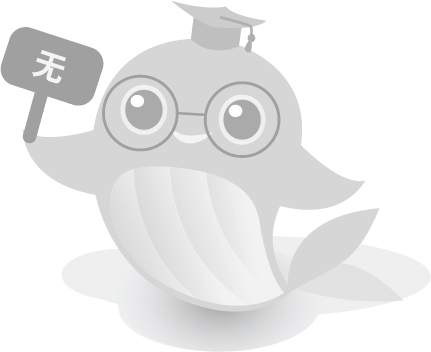
相关专栏
-
浏览量:2293次2024-01-02 16:35:00
-
浏览量:931次2023-12-06 14:42:44
-
浏览量:1195次2024-01-24 15:46:44
-
浏览量:7579次2021-03-18 08:54:25
-
浏览量:2248次2023-12-29 16:51:41
-
浏览量:1379次2024-01-24 18:44:43
-
浏览量:1784次2022-12-17 11:50:11
-
浏览量:4493次2020-09-04 15:36:49
-
浏览量:1991次2023-10-12 16:48:04
-
浏览量:1647次2020-08-19 16:35:48
-
浏览量:2639次2019-12-31 16:23:45
-
浏览量:1014次2024-01-11 15:05:58
-
浏览量:1286次2024-01-19 17:52:40
-
浏览量:3312次2020-07-29 10:00:03
-
浏览量:1098次2025-01-05 00:30:11
-
浏览量:1716次2023-10-12 14:39:21
-
浏览量:3259次2020-07-30 10:40:24
-
浏览量:1110次2023-11-24 16:31:45
-
浏览量:1798次2020-08-10 19:19:56
置顶时间设置
结束时间
删除原因
-
广告/SPAM
-
恶意灌水
-
违规内容
-
文不对题
-
重复发帖
打赏作者

merz
您的支持将鼓励我继续创作!
打赏金额:
¥1

¥5

¥10

¥50

¥100

支付方式:

举报反馈
举报类型
- 内容涉黄/赌/毒
- 内容侵权/抄袭
- 政治相关
- 涉嫌广告
- 侮辱谩骂
- 其他
详细说明
审核成功
发布时间设置
发布时间:
请选择发布时间设置
是否关联周任务-专栏模块
审核失败
失败原因
请选择失败原因
备注
请输入备注